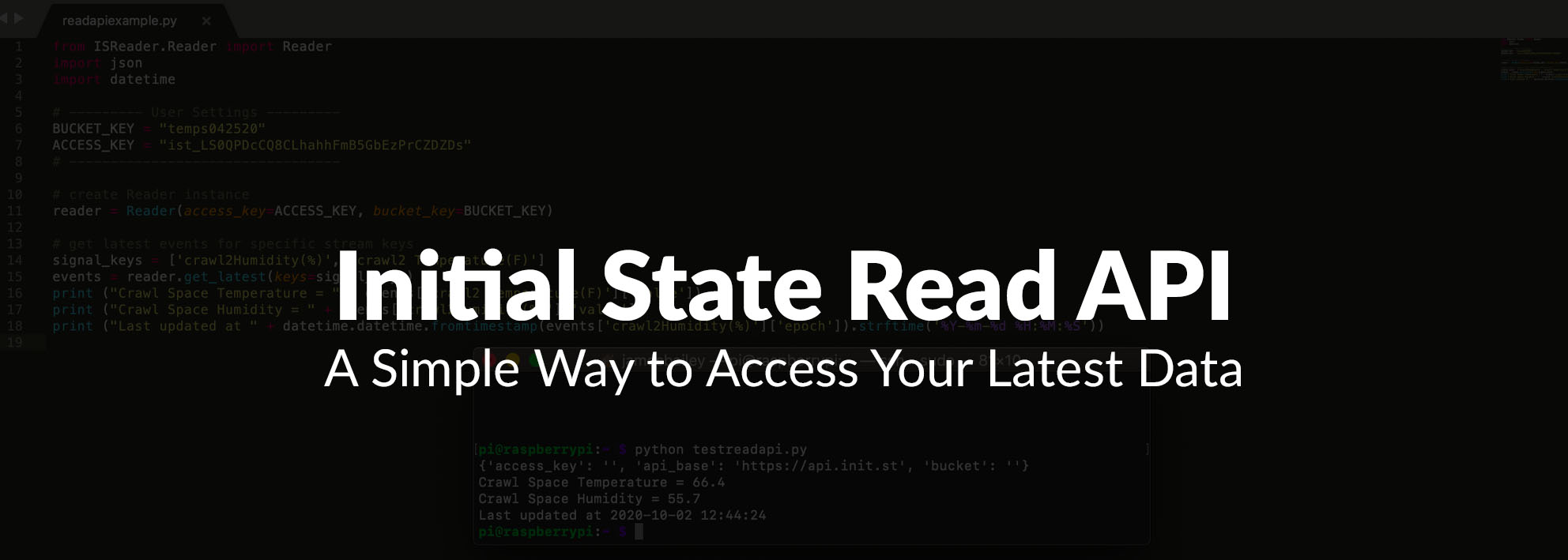
Initial State Read API:
A Simple Way to Access Your Latest Data
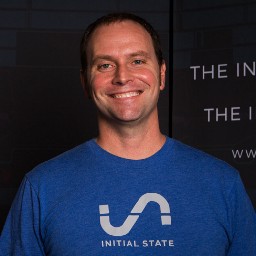
Jamie Bailey | Oct 6, 2020
Introduction
Initial State has always had an incredibly easy-to-use API for streaming data into your account. What has been missing is a similar API for reading data out of your account. That gap has now been filled with the release of the Initial State Read API. You can now enable read access to any of your data buckets and read the latest value from any data stream with a single API call. We have also released a new Python module to make accessing the Read API even easier. Let's go through some examples to show you just how easy this is to use.
If you just want to skip the examples and get right to the Read API docs, go to https://initialstatereadapi.docs.apiary.io/#.
Enabling Read API Access
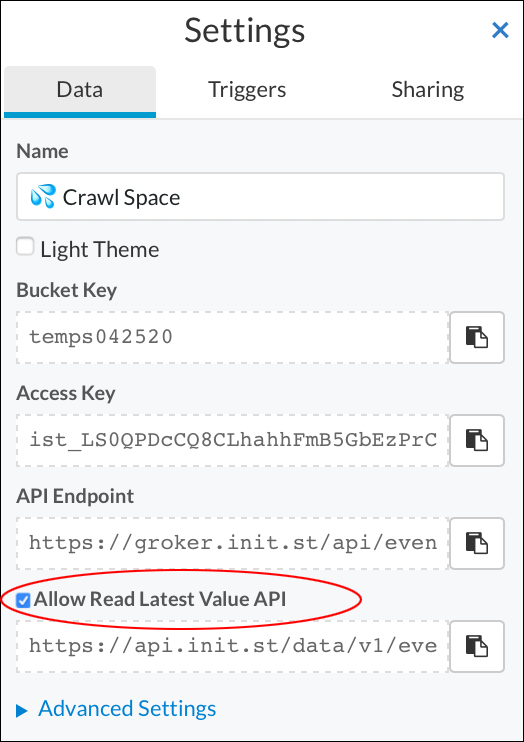
By default, read access is disabled for each of your data buckets. To enable read access, go into your bucket settings and click the Allow Read Latest Value API check box. Once enabled, the text field beneath this check box will give you your Read API endpoint URL. This URL will look something like this:
https://api.init.st/data/v1/events/latest?accessKey=YOUR_ACCESS_KEY&bucketKey=YOUR_BUCKET_KEY
- https://api.init.st/data/v1/events/latest is the base API endpoint URL.
- accessKey is the same key used for streaming data into your account (more info).
- bucketKey is the unique identifier for a specific data bucket (more info).
Testing the Read API
Testing the Read API is as simple as pasting the provided URL into the address bar of your web browser and hitting return. This will perform an HTTPS GET on the endpoint. By default, the latest value for every data stream in your data bucket will be returned along with the timestamp in epoch. For example, pasting a read URL from one of my data buckets into my Chrome browser returns the following JSON (*note, I used a JSON formatter to make the response more readable):
{
"Attic Temperature Monitor":{
"value":"Running",
"epoch":1601672453.418814
},
"Attic Temperature Monitor IP Address":{
"value":"192.168.86.32",
"epoch":1601672453.453108
},
"attic Temperature(F)":{
"value":"66.5",
"epoch":1601672925.646072
},
"atticHumidity(%)":{
"value":"44.2",
"epoch":1601672925.646869
}
}
The name of the data stream, its latest value, and the epoch timestamp are all returned in a nicely packaged JSON object.
If you want to return data from only a specified data stream instead of all data streams, you can use another URL parameter, &key=STREAM_KEY. For example, if I want to only return the latest data value from attic Temperature(F), my URL will look like the following:
https://api.init.st/data/v1/events/latest?accessKey=ist_1234&bucketKey=bkt_1234&key=attic%20Temperature(F)
The response from pasting this URL into my browser's address bar is as follows:
{
"attic Temperature(F)":{
"value":"66.5",
"epoch":1601672925.646072
}
}
You can add as many &key=STREAM_KEY stream key parameters as you need to return multiple, specific stream keys. For example:
https://api.init.st/data/v1/events/latest?accessKey=ist_1234&bucketKey=bkt_1234&key=stream_name1&key=stream_name2
"Attic Temperature Monitor":{
"value":"Running",
"epoch":1601672453.418814
},
"Attic Temperature Monitor IP Address":{
"value":"192.168.86.32",
"epoch":1601672453.453108
},
"attic Temperature(F)":{
"value":"66.5",
"epoch":1601672925.646072
},
"atticHumidity(%)":{
"value":"44.2",
"epoch":1601672925.646869
}
}
"attic Temperature(F)":{
"value":"66.5",
"epoch":1601672925.646072
}
}
Python Script Example
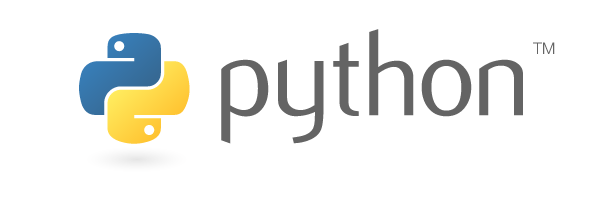
We built a Python module to make interfacing the Read API as easy as possible when writing Python applications. The source code for this module can be found at https://github.com/initialstate/python_reader. You can install this module using pip (or pip3 if you are using Python 3):
sudo pip install ISReader
or for Python 3
sudo pip3 install ISReader
Once installed, you can utilize the ISReader module to read data from a specific data stream in your data bucket. Below is an example script that reads the latest data from a single data stream then formats and prints the results:
from ISReader.Reader import Reader
import json
import datetime
# --------- User Settings ---------
ACCESS_KEY = "PLACE YOUR INITIAL STATE ACCESS KEY HERE"
BUCKET_KEY = "PLACE YOUR BUCKET KEY HERE"
STREAM_KEY = "PLACE YOUR STREAM NAME HERE"
# ---------------------------------
# create Reader instance
reader = Reader(access_key=ACCESS_KEY, bucket_key=BUCKET_KEY)
# get latest events for a specific stream key
signal_keys = [STREAM_KEY]
events = reader.get_latest(keys=signal_keys)
# format and print the resulting data and timestamp
print (STREAM_KEY + " = " + events[STREAM_KEY]['value'])
print ("Last updated at " + datetime.datetime.fromtimestamp(events[STREAM_KEY]['epoch']).strftime('%Y-%m-%d %H:%M:%S'))
You need to specify the three keys in the User Settings section. The STREAM_KEY will be the name of the data stream you want to read. The data returned by the reader.get_latest() method will be a JSON object, which makes it easy to index when importing the json module. The last line in this Python script converts the time in epoch to something a bit more human readable. Running this script on real data will produce output similar to the following:
$ python testreadapi.py
attic Temperature(F) = 66.1
Last updated at 2020-10-02 18:28:51
If you want to return data from multiple data streams, you can specify multiple signal_keys as follows:
signal_keys = ["stream_name1", "stream_name2"]
If you want to return the latest data from all data streams, you can use the following method:
all_latest_events = reader.get_latest()
import json
import datetime
# --------- User Settings ---------
ACCESS_KEY = "PLACE YOUR INITIAL STATE ACCESS KEY HERE"
BUCKET_KEY = "PLACE YOUR BUCKET KEY HERE"
STREAM_KEY = "PLACE YOUR STREAM NAME HERE"
# ---------------------------------
# create Reader instance
reader = Reader(access_key=ACCESS_KEY, bucket_key=BUCKET_KEY)
# get latest events for a specific stream key
signal_keys = [STREAM_KEY]
events = reader.get_latest(keys=signal_keys)
# format and print the resulting data and timestamp
print (STREAM_KEY + " = " + events[STREAM_KEY]['value'])
print ("Last updated at " + datetime.datetime.fromtimestamp(events[STREAM_KEY]['epoch']).strftime('%Y-%m-%d %H:%M:%S'))
attic Temperature(F) = 66.1
Last updated at 2020-10-02 18:28:51
Streaming and Reading in Python
The existing ISStreamer module makes it simple to stream data into your Initial State account when working in Python (more info). The following example script streams a random number into a data bucket then reads it back out through the Read API using the ISStreamer and ISReader Python modules.
*Make sure you enable Read API access to the specified data bucket in the UI or the reader part of this script will fail.
from ISReader.Reader import Reader
from ISStreamer.Streamer import Streamer
import json
import datetime
import random
# --------- User Settings ---------
BUCKET_KEY = "rand1234"
ACCESS_KEY = "PLACE YOUR INITIAL STATE ACCESS KEY HERE"
# ---------------------------------
# create Streamer and Reader instances
streamer = Streamer(access_key=ACCESS_KEY, bucket_key=BUCKET_KEY)
reader = Reader(access_key=ACCESS_KEY, bucket_key=BUCKET_KEY)
# stream a random value into the data bucket
streamer.log("RandomNumber", random.randint(1,1000))
# read the value from the data bucket
events = reader.get_latest(keys=['RandomNumber'])
print ("Random Number = " + events['RandomNumber']['value'])
print ("Last updated at " + datetime.datetime.fromtimestamp(events['RandomNumber']['epoch']).strftime('%Y-%m-%d %H:%M:%S'))
The output of this script will be similar to the following:
$ python streamthenread.py
Random Number = 288
Last updated at 2020-10-02 19:07:16
from ISStreamer.Streamer import Streamer
import json
import datetime
import random
# --------- User Settings ---------
BUCKET_KEY = "rand1234"
ACCESS_KEY = "PLACE YOUR INITIAL STATE ACCESS KEY HERE"
# ---------------------------------
# create Streamer and Reader instances
streamer = Streamer(access_key=ACCESS_KEY, bucket_key=BUCKET_KEY)
reader = Reader(access_key=ACCESS_KEY, bucket_key=BUCKET_KEY)
# stream a random value into the data bucket
streamer.log("RandomNumber", random.randint(1,1000))
# read the value from the data bucket
events = reader.get_latest(keys=['RandomNumber'])
print ("Random Number = " + events['RandomNumber']['value'])
print ("Last updated at " + datetime.datetime.fromtimestamp(events['RandomNumber']['epoch']).strftime('%Y-%m-%d %H:%M:%S'))
Random Number = 288
Last updated at 2020-10-02 19:07:16